React 19 Is Stable, Heres What is New!
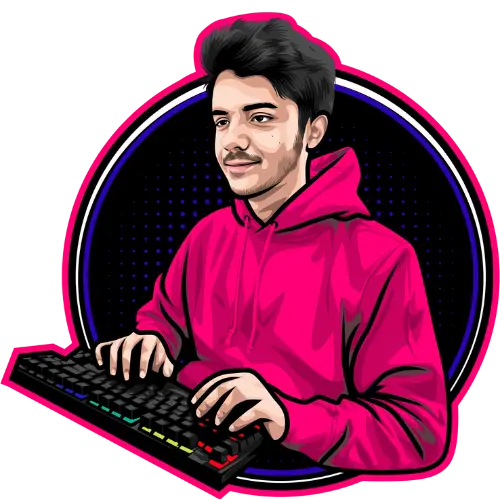
Pedro Machado / February 21, 2025
4 min read •
Description
An overview of the new features in React 19—from streamlined data mutations with Actions and powerful new hooks, to improved SSR and resource management.
React 19 Is Stable, Heres What is New!
React 19 is now officially stable and packed with a host of new features and improvements that simplify development and boost performance. In this article, we’ll walk through the most exciting changes—from streamlined data mutations with Actions and powerful new hooks, to improved server-side rendering (SSR) and resource management. If you’re using Next.js, many of these features will feel right at home.
Streamlined Data Mutations with Actions and New Hooks
React 19 introduces Actions which allow you to trigger async functions directly from your client components. This not only simplifies data mutations but also automatically manages pending states, error handling, and optimistic updates. For example, updating a user profile is now as simple as:
"use server";
export async function updateProfile(formData) {
const name = formData.get("name");
await new Promise((resolve) => setTimeout(resolve, 1500));
if (!name || name.trim() === "") {
return "Name is required.";
}
console.log("Profile updated:", name);
return null;
}
On the client side, you can consume this server action with the new hooks:
"use client";
import React from "react";
import { useActionState, useOptimistic } from "react";
import { updateProfile } from "./serverActions";
export default function ProfileForm() {
const [optimisticName, setOptimisticName] = useOptimistic("John Doe");
const [error, submitAction, isPending] = useActionState(
async (prevState, formData) => {
const newName = formData.get("name");
setOptimisticName(newName);
const errorMsg = await updateProfile(formData);
return errorMsg;
},
null
);
return (
<form action={submitAction}>
<p>Your name is: {optimisticName}</p>
<input
type="text"
name="name"
placeholder="Enter new name"
defaultValue={optimisticName}
/>
<button type="submit" disabled={isPending}>
{isPending ? "Updating..." : "Update Profile"}
</button>
{error && <p style={{ color: "red" }}>Error: {error}</p>}
</form>
);
}
Improved SSR and Resource Management
React 19 offers significant improvements for server-side rendering and resource management. New static APIs, such as prerender
, wait for data to load before streaming HTML, ensuring a smoother initial load. For example:
import { prerender } from "react-dom/static";
import App from "./App";
export async function handleRequest(request) {
const { prelude } = await prerender(<App />, {
bootstrapScripts: ["/main.js"],
});
return new Response(prelude, {
headers: { "content-type": "text/html" },
});
}
In addition, you can now declare stylesheets and async scripts directly within your component tree. React ensures these assets are deduplicated and inserted in the proper order, while new preloading APIs like prefetchDNS
and preconnect
optimize resource loading. Document metadata elements like <title>
, <meta>
, and <link>
are automatically hoisted into the <head>
, letting you manage your app’s metadata right where you need it:
export default function BlogPost({ title, author, content, keywords }) {
return (
<article>
<title>{title}</title>
<meta name="author" content={author} />
<meta name="keywords" content={keywords.join(", ")} />
<link rel="canonical" href="https://example.com/blog-post" />
<link rel="stylesheet" href="/css/blog.css" precedence="default" />
<script async src="/js/analytics.js"></script>
<h1>{title}</h1>
<p>{content}</p>
</article>
);
}
Powerful New Hooks and Compiler Improvements
React 19 also renames useFormState
to useActionState
and introduces hooks like useOptimistic
and the new use()
hook for consuming asynchronous data. These hooks reduce boilerplate and enhance performance. Moreover, the React Compiler can automatically optimize heavy computations that you previously wrapped in useMemo
. For example:
import React from "react";
function heavyComputation(data) {
let result = 0;
for (let i = 0; i < 1000000; i++) {
result += data;
}
return result;
}
export default function MyComponent({ data }) {
const computedValue = heavyComputation(data);
return <div>Computed Value: {computedValue}</div>;
}
Note: To fully utilize these compiler optimizations in Next.js, you may need to enable the React Compiler in your Next.js configuration.
Final Thoughts
From streamlined data mutations with Actions and powerful new hooks, to improved SSR and resource management, React 19 brings a lot to love. These features not only simplify your code but also boost performance and enhance the overall developer experience. If you’re using Next.js, many of these improvements will integrate naturally into your workflow.
Happy coding!